Wed, October 10, 2007, 02:23 PM under
Orcas |
VisualStudio
BackgroundAny client developer that has seen a good Windows Presentation Foundation demo/presentation will probably be salivating at the prospect of playing with this cool technology and potentially planning in their heads how they can move their applications from Windows Forms to WPF. The fact is that you don't have to and in fact shouldn't just do that yet, despite what you may have heard from overzealous presenters. Today, WPF is a great choice for consumer applications, ideal for applications where having a WOW factor is part of the requirements and definitely the only choice for software houses that have both designers and developers working on the same projects. For the rest of us building LOB apps, Windows Forms still work great and, even better, can interop with WPF if required for specific use cases. There are many resources for bidirectional interop between WPF and WinForms as well as between WPF and Win32.
The LinksIn Visual Studio 2008 we get designer support for these scenarios. If you are in a WPF project simply drag the
WindowsFormHost control from the toolbox and point it to a WinForms UserControl. I am more interested in the reverse scenario, so in a Windows Forms project drag the
ElementHost and point it to a WPF UserControl and then you can use that WPF control (set properties/call methods, handle events etc) from your winforms. Both of the host classes are implemented in the same namespace, in the WindowsFormsIntegration.dll assembly (part of WPF).
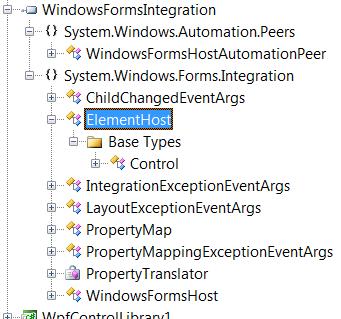
As you can see, other than the two aforementioned classes, there is a
PropertyMap class that translates properties from winforms control to WPF elements (with help from the
PropertyTranslator delegate) and you can read more about it on MSDN's aptly named
Property Mapping page. In the same namespace you can also see 4 subclasses of EventArgs (
ChildChanged,
IntegrationException,
LayoutException and
PropertyMappingException) used by events.
"Hello World"1. In VS2008, create a new WPF User Control Library project.
2. Using the designer for UserControl1.xaml create some UI or paste in the XAML section a UIElement if you already have one.
3. In the same solution, add a new Windows Forms project (in VS2008 target v3.0 or v3.5).
4. From this winforms project add a reference to the WPF User Control project
5. Rebuild all.
6. With the Form1 designer open, open the toolbox and find the UserControl1 that you created (in WPF).
7. Drag it onto the Form1, resize to your liking (it doesn't have to be docked and can coexist with WinForm controls on the form).
Notice how what it effectively did is create an
ElementHost
for you and set its
Child
property to the
UserControl1
? When it adds the
ElementHost
, it also adds references to the
WindowsFormsIntegration
,
PresentationCore
,
PresentationFramework
,
WindowsBase
and
UIAutomationProvider
assemblies. You can repeat the exercise by replacing step 7 with manually dragging an
ElementHost
onto the form and then using the ElementHost Tasks popup to select the hosted content:
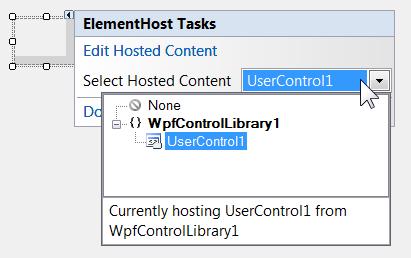
8. Ctrl+F5
9. Exercise for you dear reader: Follow the links above and see what cool sample you can come up with ;-)